Getting Started with the Vercel AI SDK: Building Powerful AI Apps
Vercel is launching new tools to improve how you work with AI
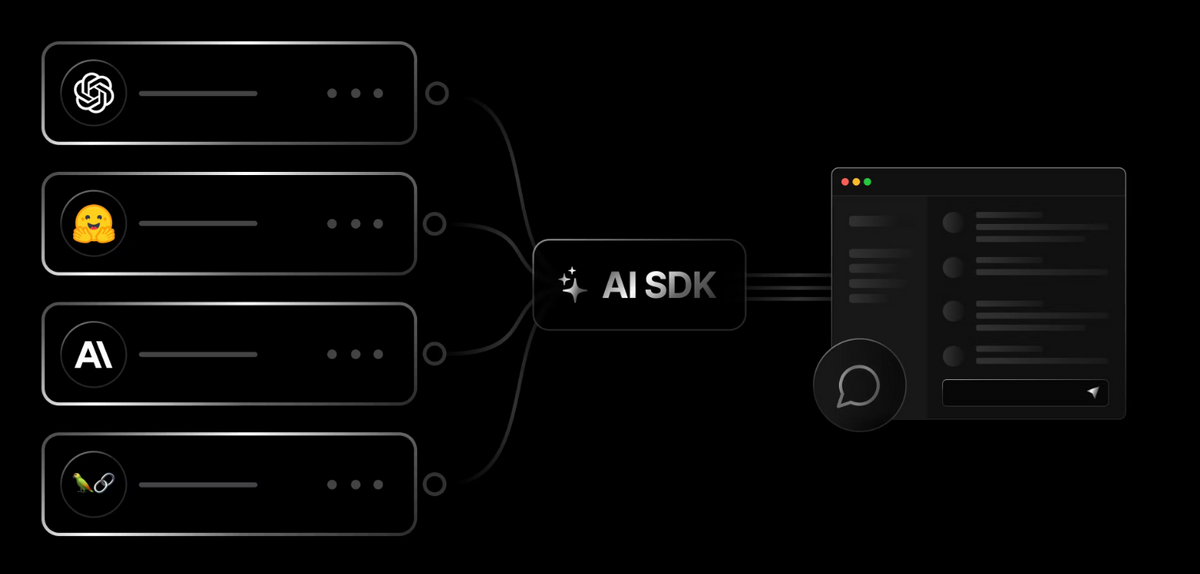
Hey there, fellow developers! Today, I want to walk you through the process of getting started with the amazing Vercel AI SDK. This open-source software development kit is designed to simplify building AI applications (like AIModels.fyi) with React and Svelte. So, let's dive in and start building some powerful AI apps!
Step 1: Installation
The first step is to install the Vercel AI SDK in your project. Open your terminal and run the following command:
npm install ai
With just that one simple command, you'll have the SDK ready to go, and you can start leveraging its powerful features right away.
Step 2: Choose Your Framework
The Vercel AI SDK supports React/Next.js and Svelte/SvelteKit. Depending on your preferred framework, choose the one that suits your project best. If you haven't already set up your chosen framework, now is the perfect time to do so. Follow the framework's documentation and create a new project or integrate the SDK into an existing project.
Step 3: Integration with AI Models
The Vercel AI SDK provides seamless integration with leading AI models such as OpenAI, LangChain, Anthropic, and Hugging Face. Let's take a look at how you can integrate these models into your application using the SDK.
Example: OpenAI Integration
To integrate OpenAI's GPT-3.5 Turbo model, follow these steps:
Import the required modules from the Vercel AI SDK and OpenAI packages:
import { OpenAIStream, StreamingTextResponse } from 'ai';
import { Configuration, OpenAIApi } from 'openai-edge';
Create an OpenAI API client using your OpenAI API key:
const config = new Configuration({
apiKey: process.env.OPENAI_API_KEY
});
const openai = new OpenAIApi(config);
Set the runtime to 'edge' to make use of Vercel's edge infrastructure:
export const runtime = 'edge';
Implement your API route or endpoint to handle requests and utilize the OpenAI model:
export async function POST(req: Request) {
const { messages } = await req.json();
const response = await openai.createChatCompletion({
model: 'gpt-3.5-turbo',
stream: true,
messages
});
const stream = OpenAIStream(response);
return new StreamingTextResponse(stream);
}
That's it! You've successfully integrated OpenAI's GPT-3.5 Turbo model into your application using the Vercel AI SDK.
Step 4: Building UI Components
Now it's time to build your AI-powered UI components. The Vercel AI SDK provides powerful React and Svelte hooks that make it a breeze to fetch and render streaming text responses. Let's take a look at an example using React:
Import the necessary hooks from the Vercel AI SDK:
import { useChat } from 'ai/react';
Set up your component and utilize the useChat
hook to handle user interactions:
export default function ChatComponent() {
const { messages, input, handleInputChange, handleSubmit } = useChat();
return (
<div>
{messages.map((message) => (
<div key={message.id}>{message.role === 'user' ? 'User: ' : 'AI: '}{message.content}</div>
))}
<form onSubmit={handleSubmit}>
<
input
value={input}
placeholder="Say something..."
onChange={handleInputChange}
/>
</form>
</div>
);
}
With just a few lines of code, you've created a chat component that utilizes the Vercel AI SDK's streaming capabilities.
Step 5: Run and Test
Now that you have integrated the Vercel AI SDK and built your UI components, it's time to run your application and test it out! Start your development server and navigate to your app in the browser. Interact with the AI-powered components and see the magic happen in real-time.
Congratulations! You've successfully started building powerful AI applications with the Vercel AI SDK. Remember to explore the SDK's documentation and examples for further inspiration and advanced usage.
Happy coding and have a fantastic time building AI-powered experiences!
Subscribe or follow me on Twitter for more content like this!
Comments ()